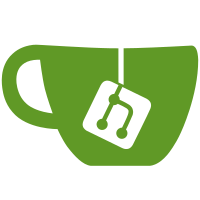
* Mute the 'stream ended' clip's audio The 'stream ended' clip plays at the at the end of every stream broadcast using owncast. It currently contains audio that peaks at -7.1db. (according to ffmpeg's volumedetect audio filter) This can result in a bad experience for viewers if the stream that they were just watching had a much lower average volume, and they had turned up their speakers or headphones to compensate. In extreme cases this could theoretically cause harm to viewers and/or their equipment. As an admin running owncast, there is no way to remove this audio *except* for patching the file. Even if you do patch the file, you need to notify your viewers to clear their browser caches if they have ever seen the clip, because offline.ts has a cache-control max-age header specifying 365 days. The caching of the previous version of this clip is out of scope of this PR. This issue is discussed in more detail in #1965. Unlike my previous attempt in #3332, which removed the audio track, this PR *mutes* the audio. Specifically, I used this ffmpeg command: ``` ffmpeg -i offline.ts -filter:a "volume=0.0" output.ts ``` There are no other modifications to the clip. * Commit updated API documentation * feat(video): make compatible muted offline clip. Rename clip as a v2 so it is not cached * Fix conflict * force add new offline file --------- Co-authored-by: vivlim <vivlim@vivl.im> Co-authored-by: Owncast <owncast@owncast.online> Co-authored-by: Gabe Kangas <gabek@real-ity.com>
110 lines
3.3 KiB
Go
110 lines
3.3 KiB
Go
package core
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
|
|
"github.com/grafov/m3u8"
|
|
"github.com/owncast/owncast/config"
|
|
"github.com/owncast/owncast/static"
|
|
"github.com/owncast/owncast/utils"
|
|
log "github.com/sirupsen/logrus"
|
|
)
|
|
|
|
func appendOfflineToVariantPlaylist(index int, playlistFilePath string) {
|
|
existingPlaylistContents, err := os.ReadFile(playlistFilePath) // nolint: gosec
|
|
if err != nil {
|
|
log.Debugln("unable to read existing playlist file", err)
|
|
return
|
|
}
|
|
|
|
tmpFileName := fmt.Sprintf("tmp-stream-%d.m3u8", index)
|
|
atomicWriteTmpPlaylistFile, err := os.CreateTemp(config.TempDir, tmpFileName)
|
|
if err != nil {
|
|
log.Errorln("error creating tmp playlist file to write to", playlistFilePath, err)
|
|
return
|
|
}
|
|
|
|
// Write the existing playlist contents
|
|
if _, err := atomicWriteTmpPlaylistFile.Write(existingPlaylistContents); err != nil {
|
|
log.Debugln("error writing existing playlist contents to tmp playlist file", err)
|
|
return
|
|
}
|
|
|
|
// Manually append the offline clip to the end of the media playlist.
|
|
_, _ = atomicWriteTmpPlaylistFile.WriteString("#EXT-X-DISCONTINUITY\n")
|
|
// If "offline" content gets changed then change the duration below
|
|
_, _ = atomicWriteTmpPlaylistFile.WriteString("#EXTINF:8.000000,\n")
|
|
_, _ = atomicWriteTmpPlaylistFile.WriteString("offline-v2.ts\n")
|
|
_, _ = atomicWriteTmpPlaylistFile.WriteString("#EXT-X-ENDLIST\n")
|
|
|
|
if err := atomicWriteTmpPlaylistFile.Close(); err != nil {
|
|
log.Errorln(err)
|
|
}
|
|
|
|
if err := utils.Move(atomicWriteTmpPlaylistFile.Name(), playlistFilePath); err != nil {
|
|
log.Errorln("error moving temp playlist to overwrite existing one", err)
|
|
}
|
|
}
|
|
|
|
func makeVariantIndexOffline(index int, offlineFilePath string, offlineFilename string) {
|
|
playlistFilePath := fmt.Sprintf(filepath.Join(config.HLSStoragePath, "%d/stream.m3u8"), index)
|
|
segmentFilePath := fmt.Sprintf(filepath.Join(config.HLSStoragePath, "%d/%s"), index, offlineFilename)
|
|
|
|
if err := utils.Copy(offlineFilePath, segmentFilePath); err != nil {
|
|
log.Warnln(err)
|
|
}
|
|
|
|
if _, err := _storage.Save(segmentFilePath, 0); err != nil {
|
|
log.Warnln(err)
|
|
}
|
|
|
|
if utils.DoesFileExists(playlistFilePath) {
|
|
appendOfflineToVariantPlaylist(index, playlistFilePath)
|
|
} else {
|
|
createEmptyOfflinePlaylist(playlistFilePath, offlineFilename)
|
|
}
|
|
if _, err := _storage.Save(playlistFilePath, 0); err != nil {
|
|
log.Warnln(err)
|
|
}
|
|
}
|
|
|
|
func createEmptyOfflinePlaylist(playlistFilePath string, offlineFilename string) {
|
|
p, err := m3u8.NewMediaPlaylist(1, 1)
|
|
if err != nil {
|
|
log.Errorln(err)
|
|
}
|
|
|
|
// If "offline" content gets changed then change the duration below
|
|
if err := p.Append(offlineFilename, 8.0, ""); err != nil {
|
|
log.Errorln(err)
|
|
}
|
|
|
|
p.Close()
|
|
f, err := os.Create(playlistFilePath) //nolint:gosec
|
|
if err != nil {
|
|
log.Errorln(err)
|
|
}
|
|
defer f.Close()
|
|
if _, err := f.Write(p.Encode().Bytes()); err != nil {
|
|
log.Errorln(err)
|
|
}
|
|
}
|
|
|
|
func saveOfflineClipToDisk(offlineFilename string) (string, error) {
|
|
offlineFileData := static.GetOfflineSegment()
|
|
offlineTmpFile, err := os.CreateTemp(config.TempDir, offlineFilename)
|
|
if err != nil {
|
|
log.Errorln("unable to create temp file for offline video segment", err)
|
|
}
|
|
|
|
if _, err = offlineTmpFile.Write(offlineFileData); err != nil {
|
|
return "", fmt.Errorf("unable to write offline segment to disk: %s", err)
|
|
}
|
|
|
|
offlineFilePath := offlineTmpFile.Name()
|
|
|
|
return offlineFilePath, nil
|
|
}
|