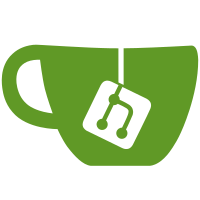
* Expand the linters and types of warnings to improve consistency and safety * Fail lint workflow if there are errors * golint has been replaced by revive * Hand-pick some of the default exclude list * Ignore error when trying to delete preview gif * Ignore linter warning opening playlist path * Rename user field Id -> ID * A bunch of renames to address linter warnings * Rename ChatClient -> Client per linter suggestion best practice * Rename ChatServer -> Server per linter suggestion best practice * More linter warning fixes * Add missing comments to all exported functions and properties
46 lines
1.2 KiB
Go
46 lines
1.2 KiB
Go
package core
|
|
|
|
import (
|
|
"github.com/owncast/owncast/config"
|
|
"github.com/owncast/owncast/core/data"
|
|
"github.com/owncast/owncast/models"
|
|
)
|
|
|
|
// GetStatus gets the status of the system.
|
|
func GetStatus() models.Status {
|
|
if _stats == nil {
|
|
return models.Status{}
|
|
}
|
|
|
|
viewerCount := 0
|
|
if IsStreamConnected() {
|
|
viewerCount = len(_stats.Viewers)
|
|
}
|
|
|
|
return models.Status{
|
|
Online: IsStreamConnected(),
|
|
ViewerCount: viewerCount,
|
|
OverallMaxViewerCount: _stats.OverallMaxViewerCount,
|
|
SessionMaxViewerCount: _stats.SessionMaxViewerCount,
|
|
LastDisconnectTime: _stats.LastDisconnectTime,
|
|
LastConnectTime: _stats.LastConnectTime,
|
|
VersionNumber: config.VersionNumber,
|
|
StreamTitle: data.GetStreamTitle(),
|
|
}
|
|
}
|
|
|
|
// GetCurrentBroadcast will return the currently active broadcast.
|
|
func GetCurrentBroadcast() *models.CurrentBroadcast {
|
|
return _currentBroadcast
|
|
}
|
|
|
|
// setBroadcaster will store the current inbound broadcasting details.
|
|
func setBroadcaster(broadcaster models.Broadcaster) {
|
|
_broadcaster = &broadcaster
|
|
}
|
|
|
|
// GetBroadcaster will return the details of the currently active broadcaster.
|
|
func GetBroadcaster() *models.Broadcaster {
|
|
return _broadcaster
|
|
}
|