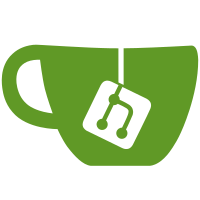
* validate json responses * update deps * tmp disable header check * log all the webfinger fails refactor and filter more malformed requests * don't set incorrect serverURL strings * test failing through admin api * fix server url in fedi tests * check response.text * validate json/xml response of all apis test Content-Type of api response and cleanup * improve logs * fix rebase * cleanup json parser in api tests * mark the api tests performed by admin * Separate check for reading and format of serverURL * test /federation/user/ with wrong username in ci
71 lines
1.5 KiB
JavaScript
71 lines
1.5 KiB
JavaScript
var request = require('supertest');
|
|
request = request('http://127.0.0.1:8080');
|
|
|
|
const defaultAdminPassword = 'abc123';
|
|
|
|
async function getAdminResponse(endpoint, adminPassword = defaultAdminPassword) {
|
|
const url = '/api/admin/' + endpoint;
|
|
const res = request
|
|
.get(url)
|
|
.auth('admin', adminPassword)
|
|
.expect(200);
|
|
|
|
return res;
|
|
}
|
|
|
|
async function sendAdminRequest(
|
|
endpoint,
|
|
value,
|
|
adminPassword = defaultAdminPassword
|
|
) {
|
|
const url = '/api/admin/' + endpoint;
|
|
const res = await request
|
|
.post(url)
|
|
.auth('admin', adminPassword)
|
|
.send({ value: value })
|
|
.expect(200);
|
|
|
|
expect(res.body.success).toBe(true);
|
|
return res;
|
|
}
|
|
|
|
async function sendAdminPayload(
|
|
endpoint,
|
|
payload,
|
|
adminPassword = defaultAdminPassword
|
|
) {
|
|
const url = '/api/admin/' + endpoint;
|
|
const res = await request
|
|
.post(url)
|
|
.auth('admin', adminPassword)
|
|
.send(payload)
|
|
.expect(200);
|
|
|
|
expect(res.body.success).not.toBe(false);
|
|
|
|
return res;
|
|
}
|
|
|
|
async function failAdminRequest(
|
|
endpoint,
|
|
value,
|
|
adminPassword = defaultAdminPassword,
|
|
responseCode = 400
|
|
) {
|
|
const url = '/api/admin/' + endpoint;
|
|
const res = await request
|
|
.post(url)
|
|
.auth('admin', adminPassword)
|
|
.send({ value: value })
|
|
.expect(responseCode);
|
|
|
|
expect(res.body.success).toBe(false);
|
|
return res;
|
|
}
|
|
|
|
module.exports.getAdminResponse = getAdminResponse;
|
|
module.exports.sendAdminRequest = sendAdminRequest;
|
|
module.exports.sendAdminPayload = sendAdminPayload;
|
|
module.exports.failAdminRequest = failAdminRequest;
|
|
|