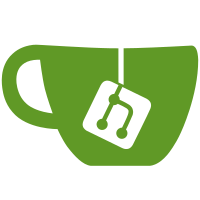
* Add emoji-mart deps * Change EmojiPicker to use emoji-mart * Change ChatTextField to work with the emoji-mart data object * Remove picmo, commit package-lock * Fix mutant svgs having a size of 0 * Get the custom emojis to show up earlier in the picker * Set emoji-mart to exact semver. Later versions break custom category sorting.
45 lines
1.3 KiB
TypeScript
45 lines
1.3 KiB
TypeScript
import React, { FC, useEffect, useState } from 'react';
|
|
import Picker from '@emoji-mart/react';
|
|
import data from '@emoji-mart/data';
|
|
|
|
export type EmojiPickerProps = {
|
|
onEmojiSelect: (emoji) => void;
|
|
customEmoji: any[];
|
|
};
|
|
|
|
export const EmojiPicker: FC<EmojiPickerProps> = ({ onEmojiSelect, customEmoji }) => {
|
|
const [custom, setCustom] = useState({});
|
|
const categories = [
|
|
'frequent',
|
|
'custom', // same id as in the setCustom call below
|
|
'people',
|
|
'nature',
|
|
'foods',
|
|
'activity',
|
|
'places',
|
|
'objects',
|
|
'symbols',
|
|
'flags',
|
|
];
|
|
// Recreate the emoji picker when the custom emoji changes.
|
|
useEffect(() => {
|
|
const e = customEmoji.map(emoji => ({
|
|
id: emoji.name,
|
|
name: emoji.name,
|
|
skins: [{ src: emoji.url }],
|
|
}));
|
|
|
|
setCustom([{ id: 'custom', name: 'Custom', emojis: e }]);
|
|
|
|
// hack to make the picker work with viewbox only svgs, 24px is default size
|
|
const shadow = document.querySelector('em-emoji-picker').shadowRoot;
|
|
const pickerStyles = new CSSStyleSheet();
|
|
pickerStyles.replaceSync('.emoji-mart-emoji {width: 24px;}');
|
|
shadow.adoptedStyleSheets = [pickerStyles];
|
|
}, []);
|
|
|
|
return (
|
|
<Picker data={data} custom={custom} onEmojiSelect={onEmojiSelect} categories={categories} />
|
|
);
|
|
};
|