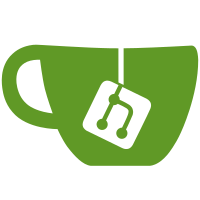
Added arguments command and label to all command executor functions, hopefully for massive performance increase. This is due to the following mess in PythonLoader: ``` Java @Override public boolean onCommand(CommandSender sender, Command command, String label, String[] args) { boolean result; if (argcount == -1) { try { result = call(4, sender, command, label, args); argcount = 4; } catch (PyException e) { //this could goof up someone ... they'll probably yell at us and eventually read this code ... fuck them if (e.type == Py.TypeError && (e.value.toString().endsWith("takes exactly 3 arguments (4 given)") || e.value.toString().endsWith("takes exactly 4 arguments (5 given)"))) { result = call(3, sender, command, label, args); argcount = 3; } else if (e.type == Py.TypeError && (e.value.toString().endsWith("takes exactly 2 arguments (4 given)") || e.value.toString().endsWith("takes exactly 3 arguments (5 given)"))) { result = call(2, sender, command, label, args); argcount = 2; } else { throw e; } } } else { result = call(argcount, sender, command, label, args); } return result; } ``` Note: Still WIP on reports - I'm working on making it keep solved reports to fix issue 10
68 lines
2.6 KiB
Python
68 lines
2.6 KiB
Python
"""
|
|
Code that was used once to create this awesome screenshot
|
|
- https://i.imgur.com/v4wg5kl.png
|
|
- https://i.imgur.com/tIZ3jmC.png
|
|
- https://www.reddit.com/r/Minecraft/comments/28le52/screenshot_of_all_players_that_joined_my_server/
|
|
"""
|
|
import net.minecraft.server.v1_7_R1.EntityPlayer as EntityPlayer
|
|
import net.minecraft.server.v1_7_R1.PacketPlayOutNamedEntitySpawn as PacketPlayOutNamedEntitySpawn
|
|
import net.minecraft.server.v1_7_R1.PlayerInteractManager as PlayerInteractManager
|
|
import net.minecraft.util.com.mojang.authlib.GameProfile as GameProfile
|
|
import org.bukkit.Bukkit as bukkit
|
|
|
|
|
|
# players.txt contains 1 name per line
|
|
players = [line.strip() for line in open("players.txt").readlines()]
|
|
|
|
ground = 70 # sea level
|
|
shift = 1.625 # amount of blocks to add on each side per row (this is for FOV 90)
|
|
amount = 6 # amount of players in first row
|
|
margin = 1 # space between players
|
|
row = 1 # distance to first row of players
|
|
goup = 6 # after how many rows should we go up by one?
|
|
upmul = 0.95 # multiplicate with goup each row
|
|
|
|
|
|
def spawn(dispname, sender, x, y, z):
|
|
"""
|
|
Sends the actual player to sender
|
|
"""
|
|
server = bukkit.getServer().getServer()
|
|
world = server.getWorldServer(0) # main world
|
|
profile = GameProfile(dispname, dispname) # set player details
|
|
manager = PlayerInteractManager(world)
|
|
entity = EntityPlayer(server, world, profile, manager) # create Player's entity
|
|
entity.setPosition(x, y, z)
|
|
packet = PacketPlayOutNamedEntitySpawn(entity) # create packet for entity spawn
|
|
sender.getHandle().playerConnection.sendPacket(packet) # send packet
|
|
|
|
|
|
@hook.command("spawnplayer")
|
|
def on_spawnplayer_command(sender, command, label, args):
|
|
global amount, row, ground, goup
|
|
|
|
# X and Z position
|
|
xpos = sender.getLocation().add(-float(row-1 * shift + (amount * margin) / 2), 0, 0).getX()
|
|
row = sender.getLocation().add(0, 0, -row).getZ()
|
|
|
|
count = 0
|
|
stop = False
|
|
while not stop:
|
|
for i in range(amount):
|
|
player = players[count]
|
|
x = int(xpos + i*margin)
|
|
spawn(player, sender, x, ground, row)
|
|
print(player, x, ground, row)
|
|
count += 1
|
|
if count >= len(players):
|
|
stop = True
|
|
print "breaking"
|
|
break
|
|
print("next row")
|
|
row -= 1 # next row (-z)
|
|
xpos -= shift # shift left
|
|
amount += int(shift*margin*2) # add players left and right
|
|
if abs(row) % int(goup) == 0:
|
|
goup *= upmul
|
|
ground += 1
|
|
print "Going up by 1: %s" % ground |